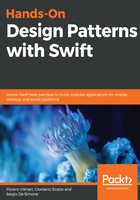
上QQ阅读APP看书,第一时间看更新
Classes
Let's start with an example of a simple class that represents a Point in an x, y coordinate system (Cartesian). Consider the following:
class Point {
var x: Double
var y: Double
init(x: Double, y: Double) {
self.x = x
self.y = y
}
}
Now, let's define a simple translate function that will mutate the x and y properties of the point objects by adding dx and dy to x and y, respectively:
func translate(point : Point, dx : Double, dy : Double) {
point.x += dx
point.y += dy
}
Now, we can create a point instance with, for example, an initial value of 0.0, and translate it to the position 1.0:
let point = Point(x: 0.0, y: 0.0)
translate(point: point, dx: 1.0, dy: 1.0)
point.x == 1.0
point.y == 1.0
Because classes follow reference semantics, only a reference to the point object is passed to the translate function; x and y are defined as var, and all of this code is valid.