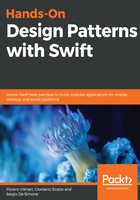
上QQ阅读APP看书,第一时间看更新
Currying
Functions and closures don't have to be defined at the top level. This can be unintuitive, when coming from languages such as Objective-C and Java. Swift, like JavaScript, lets you define functions and closures anywhere in your code. Functions can also return functions. This mechanism is known as currying.
Imagine that you want to create a logger method that will print a single argument, but it will always pretend to be a string to find it easily in your logs.
Let's start with the following basic implementation:
private let PREFIX = ‘MyPrefix'
private func log(_ value: String) {
print(PREFIX + “ “ + value)
}
class MyClass {
func doSomething() {
log(“before”)
/* complex code */
log(“after”)
}
}
While this works properly in the scope of a simple class, if you need to reuse the log method or change the internal implementation, this will lead to a lot of duplication.
You can use currying to overcome that issue, as follows:
func logger(prefix: String) -> (String) -> Void {
func log(value: String) {
print(prefix + “ “ + value)
}
return log
}
let log = logger(prefix: “MyClass”)
log(“before”)
// do something
log(“after”)
// console:
MyClass before
MyClass after