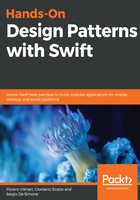
上QQ阅读APP看书,第一时间看更新
Generic functions
In Swift, the simplest form of generics would be the generics in functions. You can use generics very simply, with angled brackets, as follows:
func concat<T>(a: T, b: T) -> [T] {
return [a,b]
}
The concat method knows nothing about the types that you are passing in, but generics gives us many guarantees over using Any:
- a and b should be of the same type
- The return type is an array of elements that have the same type as a and b
- The type is inferred from the context so you don't have to type it in when you code
You can also leverage protocol conformance in your generic functions, as follows:
protocol Runnable {
func run()
}
func run<T>(runnable: T) where T: Runnable {
runnable.run()
}
In this case, the method that is run can only be called with an object that is Runnable.