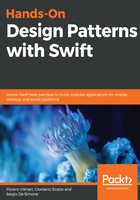
上QQ阅读APP看书,第一时间看更新
Mutability and operations
These arrays are immutable, as they are defined as let; it is therefore not possible to add or remove elements from them. Hopefully, we can still make mutable copies of them:
var otherDoubles = doubles
otherDoubles.append(4)
let lastDoubles = otherDoubles.dropFirst()
print(doubles)
print(otherDoubles)
print(lastDoubles)
Take a minute to think about what will be printed in the console, and why:
[1.0, 2.0, 3.0]
[1.0, 2.0, 3.0, 4.0]
[2.0, 3.0, 4.0]
The first array, doubles, was never mutated, as we called append(4) on a copy of it. So the copy, otherDoubles, has the value 4.0 appended to the end. Finally, the dropFirst() call returns another copy of the array, and doesn't mutate in place. This is crucial to understand these, as this behavior is consistent across all Swift Standard Library value types.