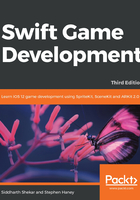
Bumping bees into bees
You may have noticed that we positioned bee2
and bee3
at the same height in the game world. We only need to push one of them horizontally to create a collision—perfect crash test dummies! We can use an impulse to create velocity for the outside bee.
Locate the didMove
function in GameScene.swift
. At the bottom, after all of our spawn code, add this line:
bee2.physicsBody?.applyImpulse(CGVector(dx: -3, dy: 0))
Run the project. You will see the outermost bee fly toward the middle and crash into the inner bee. This pushes the inner bee to the left and slows the first bee from the contact.
Attempt the same experiment with a variable: increased mass. Before the impulse line, add this code to adjust the mass of bee2
:
bee2.physicsBody?.mass = 0.2
Run the project. Hmm—our heavier bee does not move very far with the same impulse (it is a 200-gram bee, after all). It eventually bumps into the inner bee, but it is not a very exciting collision. We will need to crank up the impulse to propel our beefier bee. Change the impulse line to use a dx
value of -25
:
bee2.physicsBody?.applyImpulse(CGVector(dx: -25, dy: 0))
Run the project again. This time, our impulse provides enough energy to move the heavy bee in an interesting way. Notice how much energy the heavy bee transfers to the normal bee when they collide. Both bees possess enough momentum to eventually slide completely off the screen. Your simulator should look something like this screenshot, just before the bees slide off the screen:

Before you move on, you may wish to experiment with the various physics properties that I outlined earlier in this chapter. You can create many collision variations; the physics simulation offers a lot of depth without much effort.
Movement with impulses and forces
You have several options for moving nodes with physics bodies:
- An impulse is an immediate, one-time change to a physics body's velocity. In our test, an impulse gave the bee its velocity and it slowly bled speed to damping and its collision. Impulses are perfect for projectiles, such as missiles, bullets, disgruntled birds, and so on.
- A force applies velocity for only one physics calculation cycle. When we use a force, we typically apply it before every frame. Forces are useful for rocket ships, cars, or anything else that is continually self-propelled.
- You can also edit the
velocity
andangularVelocity
properties of a body directly. This is useful for setting a manual velocity limit.
Note
Checkpoint 3-B
The code up to this point is available in the chapter's code resources.