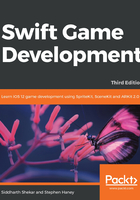
Retrofitting the Player class for flight
We need to perform a few quick setup tasks before we can react to player input. We will remove some of our older testing code and add a physics body to the Player
class.
The Beekeeper
First, clean up the old bee physics tests from the last chapter. Open GameScene.swift
, find didMove
, and locate the bottom two lines; one sets a mass for bee2
, and the other applies an impulse to bee2
. Remove these two lines:
bee2.physicsBody?.mass = 0.2 bee2.physicsBody?.applyImpulse(CGVector(dx: -25, dy: 0))
Updating the Player class
We need to give the Player
class its own update
function. We want to store player-related logic in Player
and we need it to run before every frame:
- Open
Player.swift
and add the following function insidePlayer:
func update() { }
- In
GameScene.swift
, add this code at the bottom of theGameScene
class:player.update() } override func update(_ currentTime: TimeInterval) {
- Perfect. The
GameScene
class will call thePlayer
class'supdate
function on every update.
Moving the ground
We initially placed the ground higher than necessary to make sure it displayed for all screen sizes in the previous chapter. We can now move the ground into its lower, final position since the player will soon move up and down, bringing the ground into view.
In GameScene.swift
, locate the line that sets the ground.position
value and change the y
value from 150
to 30
:
ground.position = CGPoint(x: -self.size.width * 2, y: 30)