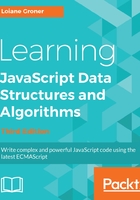
Loops
Loops are often used when we work with arrays (which are the subject of the next chapter). Specifically, we use the for loop in our algorithms.
The for loop is the same as in C and Java. It consists of a loop counter that is usually assigned a numeric value, then the variable is compared against another value (the script inside the for loop is executed while this condition is true), and finally, the numeric value is increased or decreased.
In the following example, we have a for loop. It outputs the value of i on the console, where i is less than 10; i is initiated with 0, so the following code will output the values 0 to 9:
for (var i = 0; i < 10; i++) { console.log(i); }
The next loop construct we will look at is the while loop. The block of code inside the while loop is executed while the condition is true. In the following code, we have a variable, i, initiated with the value 0, and we want the value of i to be output while i is less than 10 (or less than or equal to 9). The output will be the values from 0 to 9:
var i = 0; while (i < 10) { console.log(i); i++; }
The do...while loop is similar to the while loop. The only difference is that in the while loop, the condition is evaluated before executing the block of code, and in the do...while loop, the condition is evaluated after the block of code is executed. The do...while loop ensures that the block of code is executed at least once. The following code also outputs the values from 0 to 9:
var i = 0; do { console.log(i); i++; } while (i < 10);