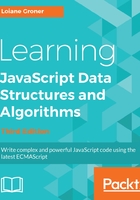
上QQ阅读APP看书,第一时间看更新
Accessing elements and iterating an array
To access a specific position of the array, we can also use brackets, passing the index of the position we would like to access. For example, let's say we want to output all the elements from the daysOfWeek array. To do so, we need to loop the array and print the elements, starting from index 0 as follows:
for (let i = 0; i < daysOfWeek.length; i++) { console.log(daysOfWeek[i]); }
Let's take a look at another example. Let's say that we want to find out the first 20 numbers of the Fibonacci sequence. The first two numbers of the Fibonacci sequence are 1 and 2, and each subsequent number is the sum of the previous two numbers:
const fibonacci = []; // {1} fibonacci[1] = 1; // {2} fibonacci[2] = 1; // {3} for (let i = 3; i < 20; i++) { fibonacci[i] = fibonacci[i - 1] + fibonacci[i - 2]; // //{4} } for (let i = 1; i < fibonacci.length; i++) { // {5} console.log(fibonacci[i]); // {6} }
The following is the explanation for the preceding code:
- In line {1}, we declared and created an array.
- In lines {2} and {3}, we assigned the first two numbers of the Fibonacci sequence to the second and third positions of the array (in JavaScript, the first position of the array is always referenced by 0 (zero), and as there is no zero in the Fibonacci sequence, we will skip it).
- Then, all we need to do is create the third to the 20th number of the sequence (as we know the first two numbers already). To do so, we can use a loop and assign the sum of the previous two positions of the array to the current position (line {4}, starting from index 3 of the array to the 19th index).
- Then, to take a look at the output (line {6}), we just need to loop the array from its first position to its length (line {5}).
We can use console.log to output each index of the array (lines {5} and {6}), or we can also use console.log(fibonacci) to output the array itself. Most browsers have a nice array representation in console.log.
If you would like to generate more than 20 numbers of the Fibonacci sequence, just change the number 20 to whatever number you like.