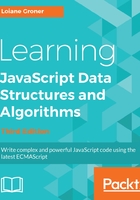
Using map and filter
JavaScript also has two other iterator methods that return a new array with a result. The first one is the map method, which is as follows:
const myMap = numbers.map(isEven);
The myMap array will have the following values: [false, true, false, true, false, true, false, true, false, true, false, true, false, true, false]. It stores the result of the isEven function that was passed to the map method. This way, we can easily know whether a number is even or not. For example, myMap[0] returns false because 1 is not even, and myMap[1] returns true because 2 is even.
We also have the filter method. It returns a new array with the elements for which the function returned true, as follows:
const evenNumbers = numbers.filter(isEven);
In our case, the evenNumbers array will contain the elements that are multiples of 2: [2, 4, 6, 8, 10, 12, 14].