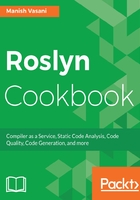
上QQ阅读APP看书,第一时间看更新
How to do it...
- In Solution Explorer, double-click on Resources.resx file in CSharpAnalyzers project to open the resource file in the resource editor.
- Replace the existing resource strings for AnalyzerDescription, AnalyzerMessageFormat and AnalyzerTitle with new strings:

- Replace the Initialize method implementation with the following:
public override void Initialize(AnalysisContext context)
{
context.RegisterSyntaxNodeAction(syntaxNodeContext =>
{
// Find implicitly typed variable declarations.
// Do not flag implicitly typed declarations that declare more than one variables,
// as the compiler already generates error CS0819 for those cases.
var declaration = (VariableDeclarationSyntax)syntaxNodeContext.Node;
if (!declaration.Type.IsVar || declaration.Variables.Count != 1)
{
return;
}
// Do not flag variable declarations with error type or special System types, such as int, char, string, and so on.
var typeInfo = syntaxNodeContext.SemanticModel.GetTypeInfo(declaration.Type, syntaxNodeContext.CancellationToken);
if (typeInfo.Type.TypeKind == TypeKind.Error || typeInfo.Type.SpecialType != SpecialType.None)
{
return;
}
// Report a diagnostic.
var variable = declaration.Variables[0];
var diagnostic = Diagnostic.Create(Rule, variable.GetLocation(), variable.Identifier.ValueText);
syntaxNodeContext.ReportDiagnostic(diagnostic);
},
SyntaxKind.VariableDeclaration);
}
- Click on Ctrl + F5 to start a new Visual Studio instance with the analyzer enabled.
- In the new Visual Studio instance, create a new C# class library with the following code:
namespace ClassLibrary
{
public class Class1
{
public void M(int param1, Class1 param2)
{
// Explicitly typed variables - do not flag.
int local1 = param1;
Class1 local2 = param2;
}
}
}
- Verify the analyzer diagnostic is not reported in the error list for explicitly typed variables.
- Now, add the following implicitly typed variable declarations to the method:
// Implicitly typed variable with error type - do not flag.
var local3 = UndefinedMethod();
// Implicitly typed variable with special type - do not flag.
var local4 = param1;
- Verify the analyzer diagnostic is not reported in the error list for implicitly typed variables with error type or special type.
- Add the violating implicitly typed variable declaration to the method:
// Implicitly typed variable with user defined type - flag.
var local5 = param2;
- Verify the analyzer diagnostic is reported for this implicitly typed variable:
