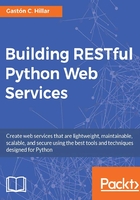
上QQ阅读APP看书,第一时间看更新
Taking advantage of generic class based views
Go to the gamesapi/games
folder and open the views.py
file. Replace the code in this file with the following code that declares the required imports and the class based views. We will add more classes to this file later. The code file for the sample is included in the restful_python_chapter_02_03
folder:
from games.models import GameCategory from games.models import Game from games.models import Player from games.models import PlayerScore from games.serializers import GameCategorySerializer from games.serializers import GameSerializer from games.serializers import PlayerSerializer from games.serializers import PlayerScoreSerializer from rest_framework import generics from rest_framework.response import Response from rest_framework.reverse import reverse class GameCategoryList(generics.ListCreateAPIView): queryset = GameCategory.objects.all() serializer_class = GameCategorySerializer name = 'gamecategory-list' class GameCategoryDetail(generics.RetrieveUpdateDestroyAPIView): queryset = GameCategory.objects.all() serializer_class = GameCategorySerializer name = 'gamecategory-detail' class GameList(generics.ListCreateAPIView): queryset = Game.objects.all() serializer_class = GameSerializer name = 'game-list' class GameDetail(generics.RetrieveUpdateDestroyAPIView): queryset = Game.objects.all() serializer_class = GameSerializer name = 'game-detail' class PlayerList(generics.ListCreateAPIView): queryset = Player.objects.all() serializer_class = PlayerSerializer name = 'player-list' class PlayerDetail(generics.RetrieveUpdateDestroyAPIView): queryset = Player.objects.all() serializer_class = PlayerSerializer name = 'player-detail' class PlayerScoreList(generics.ListCreateAPIView): queryset = PlayerScore.objects.all() serializer_class = PlayerScoreSerializer name = 'playerscore-list' class PlayerScoreDetail(generics.RetrieveUpdateDestroyAPIView): queryset = PlayerScore.objects.all() serializer_class = PlayerScoreSerializer name = 'playerscore-detail'
The following table summarizes the methods that each class-based view is going to process:

In addition, we will be able to execute the OPTIONS
HTTP verb on any of the scopes.