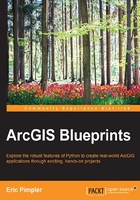
Creating the Import Collar Data tool
The following steps will help you to create Import Collar Data tool:
- Right-click on
MigrationPatterns.pyt
and select Edit. This will open your development environment, as shown in the following screenshot. Your environment will vary depending upon the editor that you defined in Chapter 1, Extracting Real-Time Wildfire Data from ArcGIS Server with the ArcGIS REST API: - Remember that you will not be changing the name of the class, which is
Toolbox
. However, you will rename theTool
class to reflect the name of the tool you want to create. - Find the class named
Tool
in your code and change the name of this tool toImportCollarData
, and set the label and description properties:class ImportCollarData(object): def __init__(self): """Define the tool (tool name is the name of the class).""" self.label = "Import Collar Data" self.description = "Import Elk Collar Data" self.canRunInBackground = False
You can use the
Tool
class as a template for other tools you'd like to add to the toolbox by copying and pasting the class and its methods. We'll do this in a later step, when we create the tool that enables the display of the elk migration patterns over time. - You will need to add each tool to the tools property (the Python list) in the
Toolbox
class. Add theImportCollarData
tool, as shown in the following code:def __init__(self): """Define the toolbox (the name of the toolbox is the name of the .pyt file).""" self.label = "Toolbox" self.alias = "" # List of tool classes associated with this toolbox self.tools = [ImportCollarData]
- Assuming that you don't have any syntax errors, you should see the following
Toolbox
orTool structure
: - In this step, we'll set the parameters for the tool. The
ImportCollarData
tool will need parameters that accept thecsv
file to be imported along with an output feature class, where the data will be written, and an input feature class to be used for schema purposes. Use thegetParameterInfo()
method to define the parameters for your tool. Individual parameter objects are created as part of this process. Add the following parameters, and then we'll discuss what the code is doing:def getParameterInfo(self): param0 = arcpy.Parameter(displayName = "CSV File to Import", \ name="fileToImport", \ datatype="DEFile", \ parameterType="Required",\ direction="Input") param1 = arcpy.Parameter(displayName = "Output Feature Class", \ name="out_fc", \ datatype="DEFeatureClass",\ parameterType="Required",\ direction="Output") param2 = arcpy.Parameter(displayName = "Schema Feature Class", \ name="schema_fc", \ datatype="DEFeatureClass",\ parameterType="Required",\ direction="Input")
Each
Parameter
object is created usingarcpy.Parameter
and is passed a number of arguments that define the object.For the first parameter object (
param0
), we are going to capture a file reference that, in this case, will be a reference to acsv
file containing the elk migration data. We give it a display name (CSV File to Import), which will be displayed on the dialog box for the tool, a name for the parameter, a datatype, a parameter type (required), and a direction.For the second parameter, we're going to define an output feature class, where the elk migration data that is read from the file will be written. Our tool will create this feature class.
The final parameter is also a feature class but has a direction of input and will be used to specify an existing feature class from which we'll pull the schema.
- Next, we'll add both the parameters to a Python list called
params
and return the list to the calling function. Add the following code:def getParameterInfo(self): param0 = arcpy.Parameter(displayName = "CSV File to Import", \ name="fileToImport", \ datatype="DEFile", \ parameterType="Required",\ direction="Input") param1 = arcpy.Parameter(displayName = "Output Feature Class", \ name="out_fc", \ datatype="DEFeatureClass",\ parameterType="Required",\ direction="Output") param2 = arcpy.Parameter(displayName = "Schema Feature Class", \ name="schema_fc", \ datatype="DEFeatureClass",\ parameterType="Required",\ direction="Input") params = [param0, param1, param2] return params