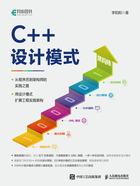
2.1.4 服装打折中的开闭原则
2.1.3小节已经完整描述了服装销售的步骤和UML类图,其中关键的类和类对应的方法也已经确定,接下来就是根据以上思路进行编程了。只要有了思路,编程是轻而易举的。为了让读者真正掌握一套程序设计的方法,在程序设计中真正做到开闭结合,下面分步来实现服装店打折的框架设计。
第一步:设计接口基类Clothes,声明派生类需要实现的虚方法。
#pragma once #include <iostream> using namespace std; #include <cstring> class Clothes { public: //服装名称 virtual string getClothesName()=0; //服装价格 virtual int getClothesPrice()=0; };
第二步:设计派生类SkirtClothes,并实现基类接口。
#include "clothes.h" //SkirtClothes派生类 class SkirtClothes:public Clothes { public: SkirtClothes(){} SkirtClothes(string _name, int _price) { this->clothesname = _name; this->clothesprice = _price; } ~SkirtClothes(){} //对基类接口函数的实现 string getClothesName(){return this->clothesname;} int getClothesPrice(){return this->clothesprice;} public: string clothesname; int clothesprice; };
第三步:设计T恤打折后的派生类OffPriceSkirtClothes。
#include "skirtclothes.h" //OffPriceSkirtClothes派生类 class OffPriceSkirtClothes:public SkirtClothes { public: //不能用初始化列表的方式(派生类不能初始化基类中的成员变量) OffPriceSkirtClothes(string _name, int _price) { this->clothesname = _name; this->clothesprice = _price; } //重写覆盖基类的实现 int getClothesPrice() { offskirtprice=SkirtClothes::getClothesPrice(); offskirtprice=offskirtprice*90/100; return offskirtprice; } private: int offskirtprice; };
第四步:新增秋季服装类AutumnClothes,并声明自身类的虚接口。
#include "clothes.h" //新增秋季服装类AutumnClothes及接口方法 class AutumnClothes:public Clothes { public: //AutumnClothes包含服装种类方法 virtual string getAutumnClothesKinds()=0; };
第五步:新增派生类SweaterAutumnClothes,实现Clothes、AutumnClothes的虚方法。
#include "autumnclothes.h" //新增派生类SweaterAutumnClothes,继承自AutumnClothes class SweaterAutumnClothes:public AutumnClothes { public: SweaterAutumnClothes(){} SweaterAutumnClothes(string _name, int _price, string _kinds) { this->clothesname = _name; this->clothesprice = _price; this->clotheskinds = _kinds; } //对基类接口函数的实现 string getClothesName(){return this->clothesname;} int getClothesPrice(){return this->clothesprice;} string getAutumnClothesKinds(){return this->clotheskinds;} public: string clothesname; int clothesprice; string clotheskinds; };
第六步:设计客户端调用类函数,遵循开闭原则。
#include "offpriceskirtclothes.h" #include "sweaterautumnclothes.h" #include <list> //一个管理所有服装类的集合 class ClothesStoreFromWenZhou { public: ClothesStoreFromWenZhou(){} ~ClothesStoreFromWenZhou(){} //获取服装类集合 void getClothes() { for (int i=0;i<3;i++) { skirt_clotheslist.push_back(b1[i]); offpriceskirt_clotheslist.push_back(b2[i]); sweaterautumn_clotheslist.push_back(b3[i]); } } //服装销售 void playClothes() { //Skirt list<SkirtClothes>::iterator item1; for (item1= skirt_clotheslist.begin(); item1!=
skirt_clotheslist.end(); item1++) { clothesname = item1->getClothesName(); clothesprice = item1->getClothesPrice(); cout<<"clothesname "<<clothesname<<" clothesprice: "<<
clothesprice<<endl; } //OffPriceSkirt list<OffPriceSkirtClothes>::iterator item2; for (item2= offpriceskirt_clotheslist.begin();
item2!=offpriceskirt_clotheslist.end(); item2++) { clothesname = item2->getClothesName(); clothesprice = item2->getClothesPrice(); cout<<"clothesname "<<clothesname<<" clothesprice: "<<
clothesprice<<endl; } //AutumnClothes list<SweaterAutumnClothes>::iterator item3; for (item3= sweaterautumn_clotheslist.begin();
item3!=sweaterautumn_clotheslist.end(); item3++) { clothesname = item3->getClothesName(); clothesprice = item3->getClothesPrice(); clotheskinds = item3->getAutumnClothesKinds(); cout<<"clothesname "<<clothesname<<" clothesprice: "<<
clothesprice<<" clotheskinds "<<clotheskinds<<endl; } } private: string clothesname; int clothesprice; //各类服装的定义及定价 list<SkirtClothes> skirt_clotheslist; SkirtClothes b1[3]={ SkirtClothes("男士Skirt",200), SkirtClothes("女士Skirt",600), SkirtClothes("小孩Skirt",100) }; list<OffPriceSkirtClothes> offpriceskirt_clotheslist; OffPriceSkirtClothes b2[3]={ OffPriceSkirtClothes("男士Skirt",200), OffPriceSkirtClothes("女士Skirt",600), OffPriceSkirtClothes("小孩Skirt",100) }; string clotheskinds; list<SweaterAutumnClothes> sweaterautumn_clotheslist; SweaterAutumnClothes b3[3]={ SweaterAutumnClothes("阿迪达斯Sweater",500,"Sweater"), SweaterAutumnClothes("李宁Sweater",600,"Sweater"), SweaterAutumnClothes("优衣库Sweater",300,"Sweater") }; }; int main() { ClothesStoreFromWenzhou clothesfromwz; clothesfromwz.getClothes(); clothesfromwz.playClothes(); return 0; }
结果显示:
clothesname男士Skirt clothesprice: 200
clothesname女士Skirt clothesprice: 600
clothesname小孩Skirt clothesprice: 100
clothesname男士Skirt clothesprice: 180
clothesname女士Skirt clothesprice: 540
clothesname小孩Skirt clothesprice: 90
clothesname阿迪达斯Sweater clothesprice: 500 clotheskinds Sweater
clothesname李宁Sweater clothesprice: 600 clotheskinds Sweater
clothesname优衣库Sweater clothesprice: 300 clotheskinds Sweater